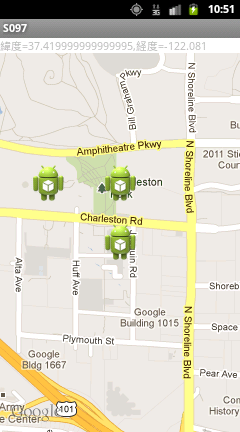
package com.efolab.s097;
import java.util.List;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Point;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.widget.LinearLayout;
import android.widget.LinearLayout.LayoutParams;
import android.widget.TextView;
import com.google.android.maps.GeoPoint;
import com.google.android.maps.MapActivity;
import com.google.android.maps.MapController;
import com.google.android.maps.MapView;
import com.google.android.maps.Overlay;
import com.google.android.maps.Projection;
public class Main extends MapActivity implements LocationListener{
Context context;
LocationManager mLocationManager;
MapController mMapController;
TextView tv;
LinearLayout base;
private final String MAP_KEY = "0HrEFkF4qrKk7s-4S8RbWvKimK2sFJHUKRAkQJQ";
MapView mapview;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
context = getApplicationContext();
base = new LinearLayout(context);
base.setOrientation(LinearLayout.VERTICAL);
setContentView(base);
mapview = new MapView(this , MAP_KEY);
mMapController = mapview.getController();
mapview.getController().setZoom(16);
mapview.setBuiltInZoomControls(true);
mapview.invalidate();
mLocationManager = (LocationManager)context.getSystemService(Context.LOCATION_SERVICE);
mLocationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 6000 , 10 , this);
tv = new TextView(context);
tv.setText("現在位置を取得しています");
base.addView(tv);
base.addView(mapview , new LinearLayout.LayoutParams(LayoutParams.MATCH_PARENT , 0 , 1.0f));
}
@Override
protected boolean isRouteDisplayed() {
TODO
return false;
}
@Override
public void onLocationChanged(Location location) {
TODO
double lat = location.getLatitude();
double lng = location.getLongitude();
GeoPoint gp = new GeoPoint((int)(lat * 1e6) , (int)(lng * 1e6));
mMapController.animateTo(gp);
tv.setText("緯度="+lat+",経度="+lng);
Bitmap icon = BitmapFactory.decodeResource(getResources(), R.drawable.ic_launcher);
AddOverlay overlay = new AddOverlay(icon , gp);
List<Overlay> overlays = mapview.getOverlays();
overlays.add(overlay);
}
private class AddOverlay extends Overlay{
Bitmap mImage;
int mOffsetX;
int mOffsetY;
GeoPoint mPoint;
AddOverlay(Bitmap image , GeoPoint initial){
mImage = image;
mOffsetX = 0 - image.getWidth() / 2;
mOffsetY = 0 - image.getHeight() / 2;
mPoint = initial;
}
@Override
public void draw(Canvas canvas, MapView mapView, boolean shadow) {
TODO
super.draw(canvas, mapView, shadow);
Projection projection = mapView.getProjection();
Point point = new Point();
projection.toPixels(mPoint , point);
point.offset(mOffsetX, mOffsetY);
canvas.drawBitmap(mImage, point.x, point.y,null);
}
}
@Override
public void onProviderDisabled(String arg0) {
TODO
}
@Override
public void onProviderEnabled(String arg0) {
TODO
}
@Override
public void onStatusChanged(String arg0, int arg1, Bundle arg2) {
TODO
}
}
Manifest.xml
<manifest xmlnsandroid="http://schemas.android.com/apk/res/android"
package="com.efolab.s097"
androidversionCode="1"
androidversionName="1.0" >
<uses-sdk
androidminSdkVersion="8"
androidtargetSdkVersion="17" />
<uses-permission androidname="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission androidname="android.permission.INTERNET"/>
<application
androidallowBackup="true"
androidicon="@drawable/ic_launcher"
androidlabel="@string/app_name"
androidtheme="@style/AppTheme" >
<activity androidname="Main">
<intent-filter>
<action androidname="android.intent.action.MAIN"/>
<category androidname="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
<uses-library androidname="com.google.android.maps"/>
</application>
</manifest>