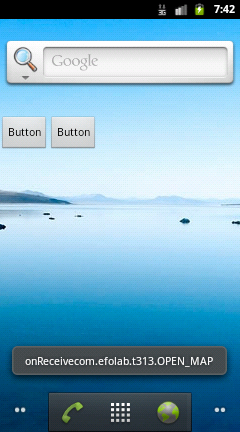
package com.efolab.t313;
import android.app.PendingIntent;
import android.appwidget.AppWidgetManager;
import android.appwidget.AppWidgetProvider;
import android.content.Context;
import android.content.Intent;
import android.widget.RemoteViews;
import android.widget.Toast;
public class MWidget extends AppWidgetProvider {
RemoteViews rv;
private final String
FILTER_MAP = "com.efolab.t313.OPEN_MAP",
FILTER_SETTING = "com.efolab.t313.OPEN_SETTING";
@Override
public void onReceive(Context context, Intent intent) {
TODO
super.onReceive(context, intent);
if(intent.getAction().equals(FILTER_MAP)){
Toast.makeText(context, "onReceive"+intent.getAction(), Toast.LENGTH_SHORT).show();
}
if(intent.getAction().equals(FILTER_SETTING)){
Toast.makeText(context, "onReceive"+intent.getAction(), Toast.LENGTH_SHORT).show();
}
}
@Override
public void onUpdate(Context context, AppWidgetManager appWidgetManager,
int[] appWidgetIds) {
TODO
super.onUpdate(context, appWidgetManager, appWidgetIds);
Toast.makeText(context, "onUpdate", Toast.LENGTH_SHORT).show();
rv = new RemoteViews(context.getPackageName(), R.layout.widget);
Intent openMap = new Intent(FILTER_MAP);
Intent openSetting = new Intent(FILTER_SETTING);
PendingIntent pendingMap = PendingIntent.getBroadcast(context, 0, openMap, 0);
PendingIntent pendingSetting = PendingIntent.getBroadcast(context, 0, openSetting, 0);
rv.setOnClickPendingIntent(R.id.button1, pendingMap);
rv.setOnClickPendingIntent(R.id.button2, pendingSetting);
appWidgetManager.updateAppWidget(appWidgetIds, rv);
}
}
Manifest.xml
<manifest xmlnsandroid="http://schemas.android.com/apk/res/android"
package="com.efolab.t313"
androidversionCode="1"
androidversionName="1.0" >
<uses-sdk
androidminSdkVersion="8"
androidtargetSdkVersion="17" />
<application
androidallowBackup="true"
androidicon="@drawable/ic_launcher"
androidlabel="@string/app_name"
androidtheme="@style/AppTheme" >
<receiver androidname="MWidget">
<intent-filter>
<action androidname="android.appwidget.action.APPWIDGET_UPDATE"/>
<action androidname="com.efolab.t313.OPEN_MAP" />
<action androidname="com.efolab.t313.OPEN_SETTING"/>
</intent-filter>
<meta-data androidname="android.appwidget.provider" androidresource="@xml/widget_info"/>
</receiver>
</application>
</manifest>
xml version="1.0" encoding="utf-8"
<appwidget-provider xmlnsandroid="http://schemas.android.com/apk/res/android"
androidinitialLayout="@layout/widget"
androidminHeight="72dp"
androidminWidth="294dp"
androidupdatePeriodMillis="0" >
</appwidget-provider>
xml version="1.0" encoding="utf-8"
<LinearLayout xmlnsandroid="http://schemas.android.com/apk/res/android"
androidlayout_width="match_parent"
androidlayout_height="match_parent"
androidorientation="horizontal" >
<Button
androidid="@+id/button1"
androidlayout_width="wrap_content"
androidlayout_height="wrap_content"
androidtext="Button" />
<Button
androidid="@+id/button2"
androidlayout_width="wrap_content"
androidlayout_height="wrap_content"
androidtext="Button" />
</LinearLayout>